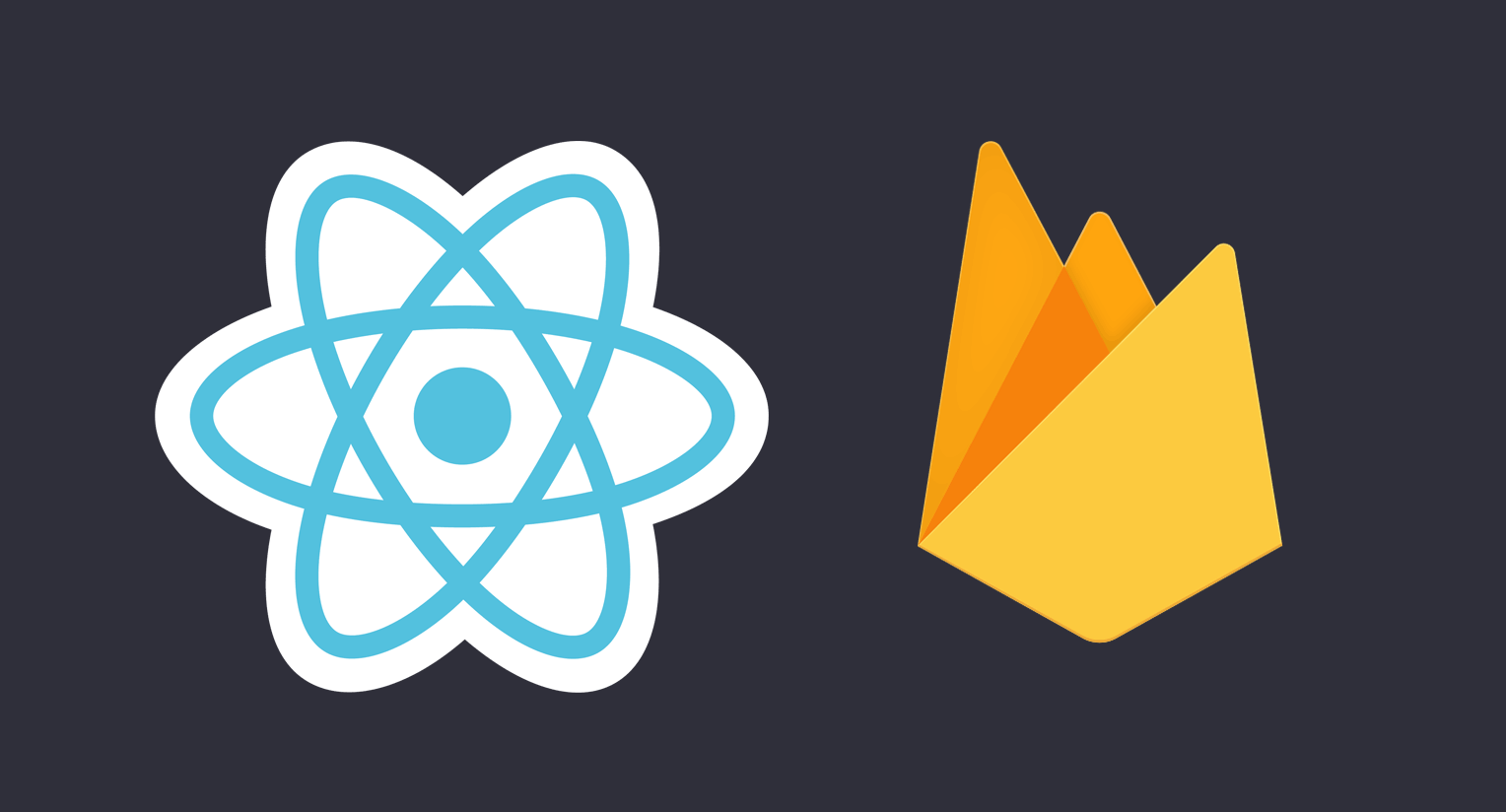
Making My First React Website (With Firebase)
Motivation
After learning the basics of web development (i.e. HTML, CSS, JavaScript) through the creation of my personal website, I knew that I wanted to extend my development capabilites through a front-end framework. This is because I enjoyed the creation of my own website, but I wanted to learn to make more dynamic pages, with more that just the ability to make a static, non-interactive site. I found that the big three options I had to choose from were ReactJS, developed by Facebook, AngularJS, developed by Google, or VueJS. I decided to learn React due to a suggestion from a friend, as well as the fact that, unlike the other options, it is simply a JavaScript library, and not an entire Model View Controller (MVC) framework. I knew that the site site I wanted to create, which I will get to shortly, would require data persistence, which would require some sort of backend. In the spirit of not overwhelming myself by trying to learn too much at one time, I decided to use Firebase for my data persistence, since it could do everything I needed, along with user authentication, and has a generous free tier, which does not require a paymnent plan unless your site grows to a certain usage level. I knew that my brother would be proposing to his girlfriend soon, so I wanted to provide him with a very personalized gift, by making them a website for their wedding, where people could RSVP, leave messages, and view engagement photos, and interact by leaving comments. Now that I knew what I wanted to do, and what tools I wanted to use to make it happen, next was for me to learn the requisite skills to get comfortable enough to start my own project.
Process
Being in the Army, one of the most frequently used phrases/models that comes up when learning any new skill is the idea of crawl -> walk -> run, which is just the practice of starting slow, and progressively working your way up to more difficult versions of the task. For this reason, when I learn something new, I try to not add too many new tools into my workflow at once, and instead take the time to really learn one at a time well. Any of the aforementioned Javascript frameworks/libraries require you to abstract your way of thinking about web design differently than just writing everything in pure HTML/CSS/JS. They require you to think in terms of components and state, which are basic concepts that must be understood in the crawl phase before you have any chance of being able to "walk". Seing as I was unfamiliar with most of these concepts, I went to YouTube to search for some intro / beginner level videos regarding React. I found a 2 hour crash course by an instructor named Mosh Hamedani, who offered 2 hours of his 15 hour React course for free on YouTube. I was pleasantly surpised by it, and really appreciated his project-based approach to teaching, so decided to take his entire course. This course effectively served as my "crawl" phase, teaching me all of the React concepts, while also providing me with the components built during the course that I would later reuse in my website.
Now that I was relatively comfortable with understanding React (which if you are struggling with, here is a great guide from the React Development Team), I could move forward with making my own website. For this, I would need at least a cursory understanding of Firebase, its tools, and how to use them. For this, I just perused YouTube for videos of "how to integrate Firestore with ReactJS", as well as the Firebase documentation. I reference a 12-hour video tutorial series offered through Free Code Camp about making a Twitter clone using React and Firebase to get a sense of how Firebase and React would interact. I did not watch all 12 hours, but watched the first few to get a good feel of how the two technologies work together.
The website I made, which I coined WeddingBoard, used Firebase's Cloud Storage to store the photos which would be used for the photo gallery, and I used Firestore to maintain three documents: Users, Gallery, RSVPs, and Pinboard. I wanted to have three different roles for the site: anonymous (not logged in), authenticated (logged in), and admin, which would be reserved for myself and the wedding couple. The anonymous role can view everything an authenticated user can view, but cannot post anything. An authenticated user can post comments on photos, messages on the pinboard, RSVP to the wedding, as well as delete their own comments or messages. Admins can do all of the above, plus delete any comments, delete photos, and add photos. To accomplish this, I would have a global state that is kept on the App component level, which keeps track of the current user, whether the user is logged in, and whether the user is admin. This state gets updated whenever the authentication listener gets called, and this state is passed to all children (I will talk later about better ways to do this). This reinforces one of the cardinal rules of component-based frameworks, which is to have a single source of truth. In this case, the App component state is the single source of truth for all user related information.
I kept track of comments by having my Gallery document have a field for each photo in the gallery, and each photo also has an array of comments, with the user ID, the author's display name, the comment itself, and a timestamp. I kept this system simple, and this works since I do not even try to implement repying to comments/sub-threads, or editing comments. When the page loads, if the user ID of a given comment matches the user ID of the current user, React renders a 'Delete' button for the users to delete comments that they wrote. This button also always renders if the current user is an admin. I used this same general structure for all other documents in the database. React makes it very easy to implement these control flows, using state and props.
Learning Resources :
- Programming With Mosh
- ReactJS Documentation
- Firebase Documentation
- Recact Firebase Social Media App Course
Component Libraries Used :
Lessons Learned & Next Steps
All in all, it took me roughly a week of working on the site to get it to a point where I was comfortable enough to deploy it, with a few tweaks here and there after the initial deployment. Overall, I am happy with how the site turned out, although as any developer knows, it will never be perfect, especially in the eyes of the person who made it. I made some mistakes ealy on in the process, such as not having the single source of truth, or not having it high enough in the component tree, which caused some issues that I had to work through. I got experience using component libraries, and interacting with Firebase, which is a great tool for front-end development to get past the hurdles of needed a backend. Also, I learned that sometimes, what the developer thinks would be the coolest or best option, is not the best if the people who will be using the product do not want it.
I could always think of new ways to make the site more complicated and, in my eyes "cooler", but if that is not what the couple wants, or if it will cause it to take too much longer to develop, it is not as useful. Oftentimes, an imperfect good solution is better than a perfect complex and time-consuming solution. If I were to redo this site, I would think of using a library such as Redux for better state management, since, as I mentioned ealier, it can get very tedious to pass the App component state to every componeent, since it is needed thorughout most of the application. The site is by no means perfect or the best way to implement the features that I wanted, but I am happy with how it turned out, and grateful that I could make something that has a practical use.
If you would like to see the site, you can visit it here.